How to Use AWS Lambda for Building Serverless Microservices
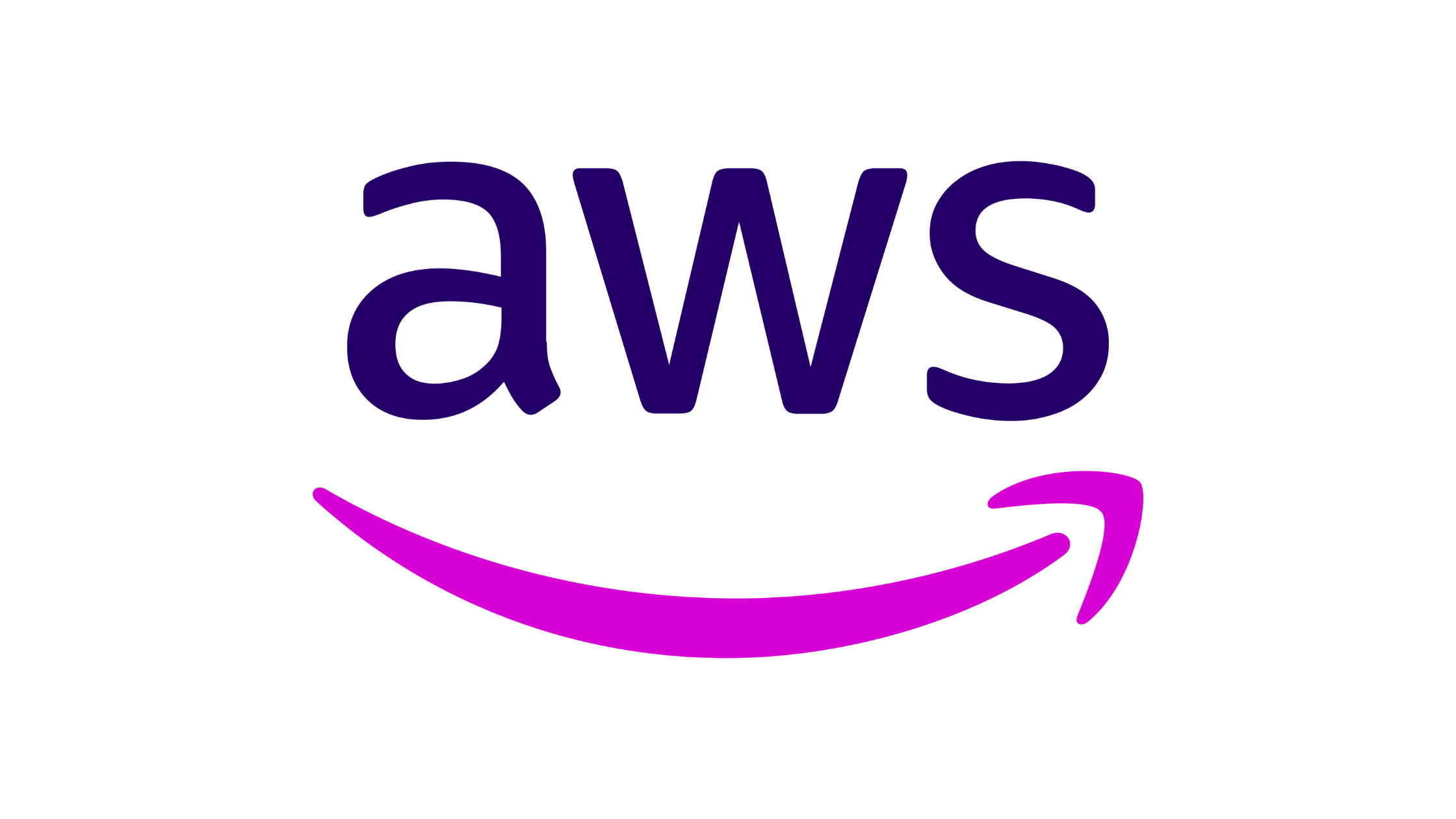
The rise of serverless computing has revolutionized how we approach software development. By eliminating the need to manage servers and infrastructure, developers can focus on building and deploying applications faster and more efficiently. AWS Lambda, a serverless compute service, stands out as a powerful tool for building microservices, offering a range of benefits and possibilities.
This article will delve into the intricacies of using AWS Lambda for building serverless microservices, providing a comprehensive guide for developers looking to leverage this technology.
Understanding Microservices and Serverless Architecture
Microservices represent a modern architectural approach where applications are broken down into smaller, independent services that communicate with each other over a network. Each microservice focuses on a specific business function, making them easier to develop, deploy, and maintain independently.
Serverless computing, on the other hand, allows developers to run code without provisioning or managing servers. This means that resources are automatically provisioned and scaled based on demand, eliminating the overhead associated with traditional server management.
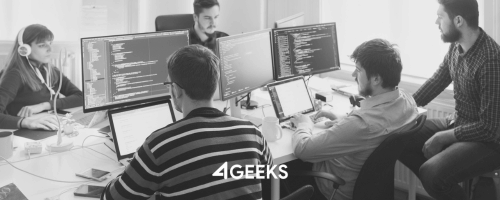
Custom Software Engineering Services
Work with our in-house Project Managers, Software Engineers and QA Testers to build your new custom software product or to support your current workflow, following Agile, DevOps and Lean methodologies.
Combining microservices with a serverless architecture using AWS Lambda offers numerous advantages:
1. Reduced Operational Costs: By eliminating the need for server management, developers can significantly reduce operational costs associated with infrastructure maintenance, scaling, and security.
2. Improved Scalability: AWS Lambda automatically scales resources based on demand, ensuring that your microservices can handle peak workloads without performance degradation.
3. Faster Development and Deployment: The serverless approach allows developers to focus on building and deploying code quickly, as they don't have to worry about infrastructure configuration.
4. Enhanced Resilience: Microservices built with Lambda are inherently resilient as they are deployed independently and can be scaled and updated without affecting other services.
Building Serverless Microservices with AWS Lambda
Let's break down the process of building serverless microservices using AWS Lambda, focusing on key concepts and best practices:
1. Define Microservice Functions:
Start by identifying the business functions you want to break down into microservices. Each microservice will be represented by a Lambda function that performs a specific task. For instance, a "user registration" microservice could handle the creation and verification of new user accounts.
2. Choose the Programming Language and Runtime:
AWS Lambda supports a wide range of programming languages, including Node.js, Python, Java, Go, and .NET Core. Select the language that best suits your project requirements and existing expertise.
3. Create Lambda Functions:
You can create Lambda functions using the AWS console, AWS CLI, or infrastructure-as-code tools like CloudFormation. When creating a function, you'll define the following:
- Function name: A unique identifier for your Lambda function.
- Runtime: The programming language and version you'll use.
- Memory: The amount of RAM allocated to your function.
- Timeout: The maximum time your function can execute before being terminated.
- Handler: The entry point for your code within your function.
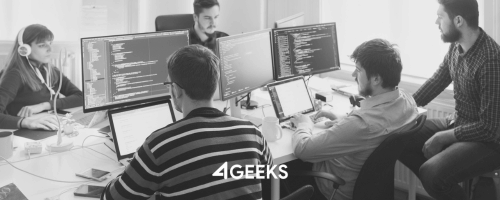
Custom Software Engineering Services
Work with our in-house Project Managers, Software Engineers and QA Testers to build your new custom software product or to support your current workflow, following Agile, DevOps and Lean methodologies.
4. Write the Function Code:
Implement the business logic for your microservice within your Lambda function. This code will handle the specific task assigned to the function, such as processing user requests, accessing databases, or interacting with other services.
5. Trigger Lambda Functions:
Lambda functions can be triggered by various events, including:
- API Gateway: Use API Gateway to expose your Lambda functions as REST APIs. This allows you to build web applications that interact with your microservices.
- S3 Events: Trigger Lambda functions when objects are uploaded or modified in Amazon S3 buckets.
- DynamoDB Streams: Execute functions in response to changes in DynamoDB tables.
- CloudWatch Events: Schedule periodic tasks or respond to system events using CloudWatch Events.
6. Handle Data and Dependencies:
Lambda functions can access and interact with various AWS services, including databases, storage services, and message queues. To ensure data consistency and security, consider using best practices like:
- Database access: Use AWS SDKs for efficient and secure interaction with databases like DynamoDB, Amazon RDS, or Amazon Redshift.
- Data storage: Store and manage data securely in services like Amazon S3.
- Message queues: Use AWS services like Amazon SQS or Amazon SNS for asynchronous communication between microservices.
7. Test and Deploy:
Thoroughly test your Lambda functions to ensure they perform correctly and meet your performance requirements. You can use AWS Lambda's built-in testing tools or integrate third-party testing frameworks. Once your functions are validated, deploy them to your chosen environment.
8. Monitor and Optimize:
Monitor your Lambda functions using CloudWatch metrics to track performance, identify potential issues, and optimize resource utilization. Regularly review your function configurations and code to ensure they are efficient and scalable.
Example: Building a "User Registration" Microservice
Let's illustrate these concepts by creating a simple "User Registration" microservice using AWS Lambda and API Gateway:
1. Define the Microservice Function:
We'll create a Lambda function named "createUser" responsible for handling user registration requests.
2. Choose Language and Runtime:
For this example, we'll use Node.js as our programming language and the latest Node.js runtime.
3. Create the Lambda Function:
Using the AWS console, create a new Lambda function with the following configuration:
- Function name: createUser
- Runtime: Node.js 18.x
- Memory: 128 MB
- Timeout: 30 seconds
- Handler: index.handler
4. Write the Function Code:
exports.handler = async (event) => {
const body = JSON.parse(event.body);
const { username, email, password } = body;
// Perform user registration logic here
// Example: Store user data in a database
return {
statusCode: 201,
body: JSON.stringify({ message: "User created successfully" }),
};
};
content_copyUse code with caution.JavaScript
5. Trigger the Function with API Gateway:
Create a new API Gateway endpoint that calls the "createUser" function when a POST request is received at a specific endpoint, for example: /users.
6. Test and Deploy:
Test your API Gateway endpoint to verify that user registration requests are handled correctly. Once satisfied, deploy your microservice to your desired environment.
Best Practices for Building Serverless Microservices with AWS Lambda
- Use the AWS SDKs: Utilize the official AWS SDKs for your chosen programming language to interact with AWS services securely and efficiently.
- Implement error handling: Implement robust error handling mechanisms to handle exceptions and ensure your microservices remain stable.
- Log and monitor: Leverage CloudWatch logs and metrics for comprehensive monitoring and debugging of your Lambda functions.
- Use serverless frameworks: Explore serverless frameworks like Serverless Framework or AWS SAM to simplify development, deployment, and management.
- Optimize for cost: Optimize your Lambda functions by using appropriate memory and timeout configurations to minimize costs.
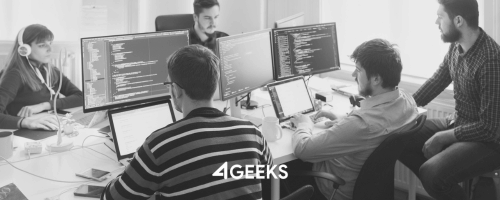
Custom Software Engineering Services
Work with our in-house Project Managers, Software Engineers and QA Testers to build your new custom software product or to support your current workflow, following Agile, DevOps and Lean methodologies.
Conclusion
By leveraging AWS Lambda and serverless principles, developers can build powerful and scalable microservices that offer numerous benefits. This approach enables faster development cycles, reduced operational costs, and enhanced resilience.
By adhering to best practices and utilizing the AWS ecosystem effectively, you can unlock the true potential of serverless microservices and create modern, efficient applications for the cloud.
FAQs
What are the cost implications of using AWS Lambda for serverless microservices?
AWS Lambda pricing is based on the number of requests and the duration of execution. The first 1 million requests per month are free, and beyond that, you are charged per request and for the compute time used, measured in milliseconds. This can lead to significant savings compared to traditional server-based approaches, especially for applications with variable workloads. However, it’s important to monitor usage and optimize function performance to control costs.
How does AWS Lambda handle state management in microservices?
AWS Lambda is inherently stateless, meaning each invocation is isolated with no knowledge of previous executions. To manage state, developers typically use external storage solutions like Amazon DynamoDB for persistent storage or Amazon S3 for storing larger datasets. For workflows requiring state persistence across multiple steps, AWS Step Functions can be used to coordinate the execution of multiple Lambda functions.
What are some common security considerations and best practices for AWS Lambda?
- IAM Roles and Policies: Use fine-grained IAM roles to grant the minimum necessary permissions to your Lambda functions.
- Environment Variables: Securely manage sensitive data using AWS Secrets Manager or AWS Systems Manager Parameter Store.
- VPC Integration: Run Lambda functions inside a VPC for enhanced network security and to access resources in private subnets.
- Monitoring and Logging: Enable AWS CloudWatch Logs for Lambda functions to track execution and detect security issues.
- Code Vulnerabilities: Regularly scan and update your Lambda function code to patch known vulnerabilities.