How to Containerize Microservices for Serverless Deployment
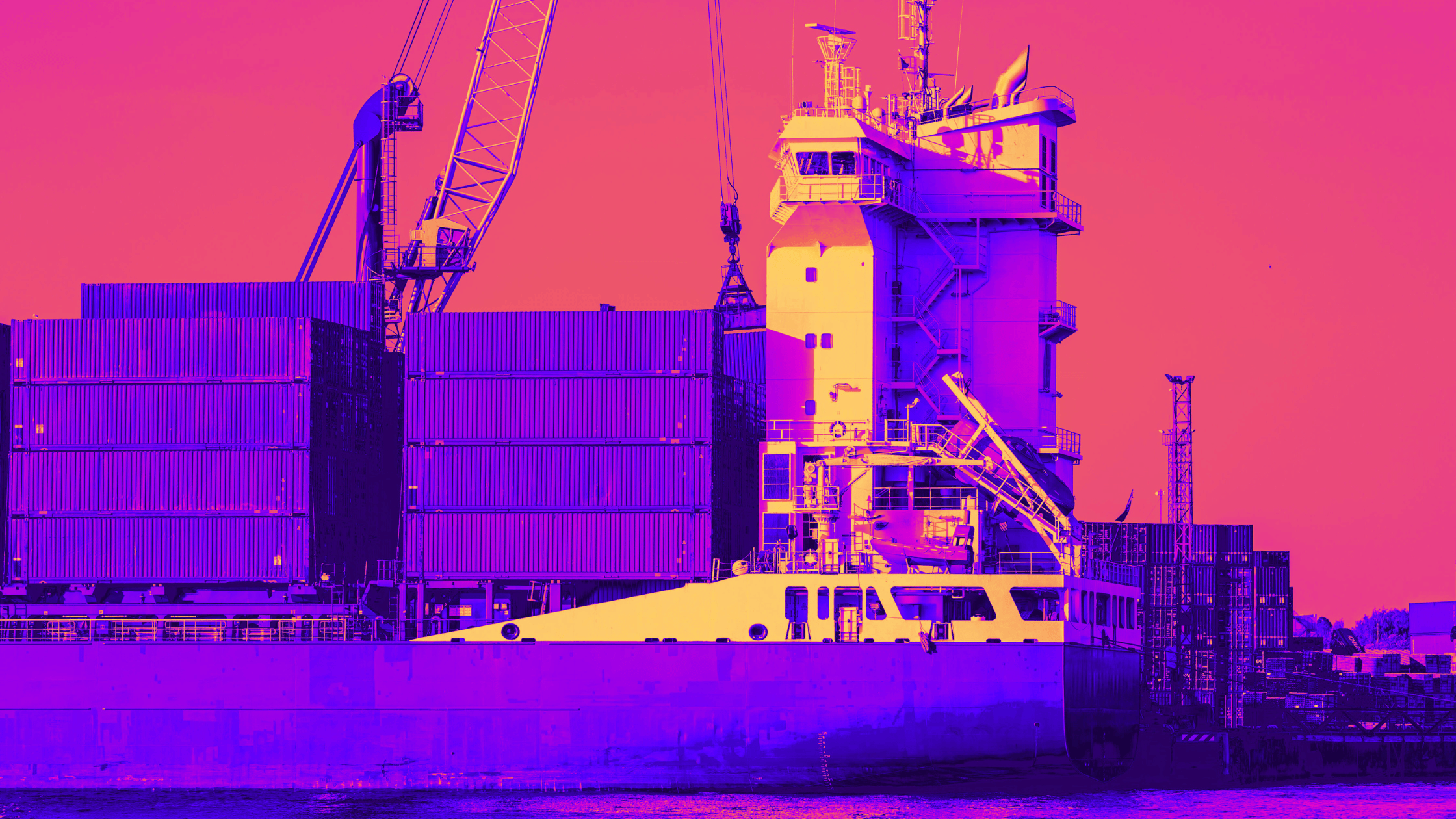
In today's dynamic software landscape, microservices have become a popular architectural style for building scalable and maintainable applications. Coupled with serverless deployment, microservices can offer unprecedented flexibility, cost-efficiency, and ease of management.
This article delves into the technical intricacies of containerizing microservices for serverless deployment, ensuring that your applications are both robust and agile.
Understanding Microservices and Serverless Architecture
Microservices
Microservices architecture involves breaking down an application into smaller, independent services that communicate through APIs. Each service is developed, deployed, and scaled independently, promoting better modularity, scalability, and fault isolation. Key characteristics of microservices include:
- Decoupled Services: Each service handles a specific business function.
- Independent Deployment: Services can be updated without redeploying the entire application.
- Technology Diversity: Different services can use different programming languages and databases.
Serverless Architecture
Serverless architecture abstracts server management away from the developer. Instead of provisioning, scaling, and managing servers, developers focus on writing code while the cloud provider handles the infrastructure. Key benefits include:
- Cost-Efficiency: Pay only for the compute time you consume.
- Auto-Scaling: Automatically scales with demand.
- Reduced Operational Overhead: No need for server maintenance or capacity planning.
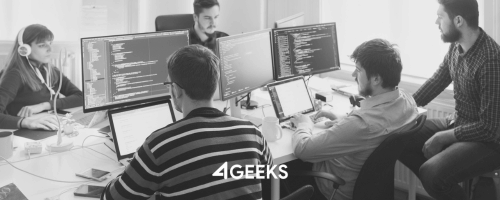
Custom Software Engineering Services
Work with our in-house Project Managers, Software Engineers and QA Testers to build your new custom software product or to support your current workflow, following Agile, DevOps and Lean methodologies.
Why Containerize Microservices for Serverless Deployment?
Combining containerization with serverless deployment offers several advantages:
- Portability: Containers ensure that applications run consistently across different environments.
- Isolation: Each microservice runs in its own container, preventing conflicts and enhancing security.
- Efficient Resource Utilization: Containers are lightweight and share the host OS kernel, leading to better resource utilization.
- Scalability: Containers can be orchestrated to scale efficiently in a serverless environment.
Steps to Containerize Microservices for Serverless Deployment
1. Design Your Microservices
Before containerizing, ensure your application is designed as microservices. Each microservice should:
- Handle a single business capability.
- Be independently deployable.
- Communicate with other services through well-defined APIs.
2. Choose the Right Containerization Tool
Docker is the most popular tool for containerization. It allows you to package applications with all their dependencies into containers.
3. Write Dockerfiles for Each Microservice
A Dockerfile is a script that contains instructions to build a Docker image. Create a Dockerfile for each microservice. Here’s an example Dockerfile for a Node.js microservice:
# Use an official Node runtime as the base image
FROM node:14
# Set the working directory
WORKDIR /usr/src/app
# Copy package.json and package-lock.json
COPY package*.json ./
# Install dependencies
RUN npm install
# Copy the rest of the application code
COPY . .
# Expose the port the app runs on
EXPOSE 8080
# Define the command to run the app
CMD ["node", "index.js"]
4. Build Docker Images
Use the docker build
command to create Docker images from the Dockerfiles. Tag the images for easy identification and versioning.
docker build -t my-microservice:1.0 .
5. Push Docker Images to a Container Registry
A container registry stores and distributes Docker images. Popular registries include Docker Hub, AWS Elastic Container Registry (ECR), and Google Container Registry (GCR).
docker tag my-microservice:1.0 myregistry/my-microservice:1.0
docker push myregistry/my-microservice:1.0
6. Configure Serverless Framework
The Serverless Framework is a powerful tool for deploying applications to serverless environments. Install the framework and create a serverless configuration file (serverless.yml
).
npm install -g serverless
serverless create --template aws-nodejs --path my-service
7. Define the Serverless Service
In serverless.yml
, configure the service to use your Docker image. Here’s an example configuration for an AWS Lambda function:
service: my-service
provider:
name: aws
runtime: nodejs14.x
functions:
myFunction:
image:
name: myregistry/my-microservice:1.0
command:
- node
- index.js
resources:
Resources:
MyLogGroup:
Type: AWS::Logs::LogGroup
Properties:
LogGroupName: /aws/lambda/my-service
RetentionInDays: 14
8. Deploy to Serverless Environment
Deploy your service using the Serverless Framework:
serverless deploy
This command packages your application, uploads it to the specified serverless provider (e.g., AWS Lambda), and creates the necessary infrastructure.
9. Monitor and Scale
Use monitoring tools to track the performance of your microservices. AWS CloudWatch, Google Cloud Monitoring, and Azure Monitor are popular choices. These tools help you:
- Monitor logs and metrics.
- Set up alerts for critical issues.
- Scale services automatically based on demand.
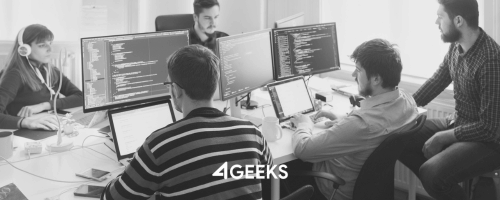
Custom Software Engineering Services
Work with our in-house Project Managers, Software Engineers and QA Testers to build your new custom software product or to support your current workflow, following Agile, DevOps and Lean methodologies.
Best Practices
Optimize Docker Images
- Use Multi-Stage Builds: Reduce image size by using multi-stage builds.
- Minimize Layers: Combine commands to minimize the number of layers.
- Use Official Base Images: Base your images on official, minimal base images.
Secure Your Containers
- Scan for Vulnerabilities: Use tools like Clair or Trivy to scan images for vulnerabilities.
- Limit Permissions: Run containers with the least privilege necessary.
- Regular Updates: Regularly update base images and dependencies.
Automate CI/CD
Implement Continuous Integration and Continuous Deployment (CI/CD) pipelines using tools like Jenkins, GitLab CI, or GitHub Actions. Automate the build, test, and deployment processes to ensure consistent and reliable deployments.
Use Infrastructure as Code (IaC)
Define and manage your infrastructure using IaC tools like Terraform, AWS CloudFormation, or Pulumi. This ensures that your infrastructure is versioned and replicable.
Conclusion
Containerizing microservices for serverless deployment marries the best of both worlds: the scalability and modularity of microservices with the flexibility and cost-efficiency of serverless architecture.
By following the steps outlined in this guide, you can build, deploy, and manage robust applications that are ready to handle modern demands. Embrace this powerful combination to elevate your application's performance and scalability.
FAQs
How do I handle database connections in a serverless environment?
Database connections in a serverless environment can be challenging due to the ephemeral nature of serverless functions, which can lead to connection exhaustion. Solutions include using database connection pooling, leveraging managed database services that support serverless (like Amazon Aurora Serverless), and employing middleware like AWS RDS Proxy, which manages connections efficiently.
What are the cost implications of using containers in serverless architectures?
While serverless architectures offer cost-efficiency by charging only for the compute time used, introducing containers can add complexity and potential costs. You need to consider the cost of container orchestration services (like AWS Fargate or Google Cloud Run) and the container registry storage fees. However, containers can lead to better resource utilization and reduced operational overhead, potentially balancing out the additional costs.
Can I run stateful applications in a serverless environment?
Running stateful applications in a serverless environment is possible but requires careful planning. Serverless functions are inherently stateless, so you need to manage state externally using services like AWS DynamoDB, Redis, or S3 for persistent storage. For more complex stateful requirements, consider using a combination of serverless and stateful services like managed Kubernetes (with StatefulSets) or hybrid architectures that blend serverless functions with stateful backend services.