How to Integrate Serverless Functions with Existing Microservices
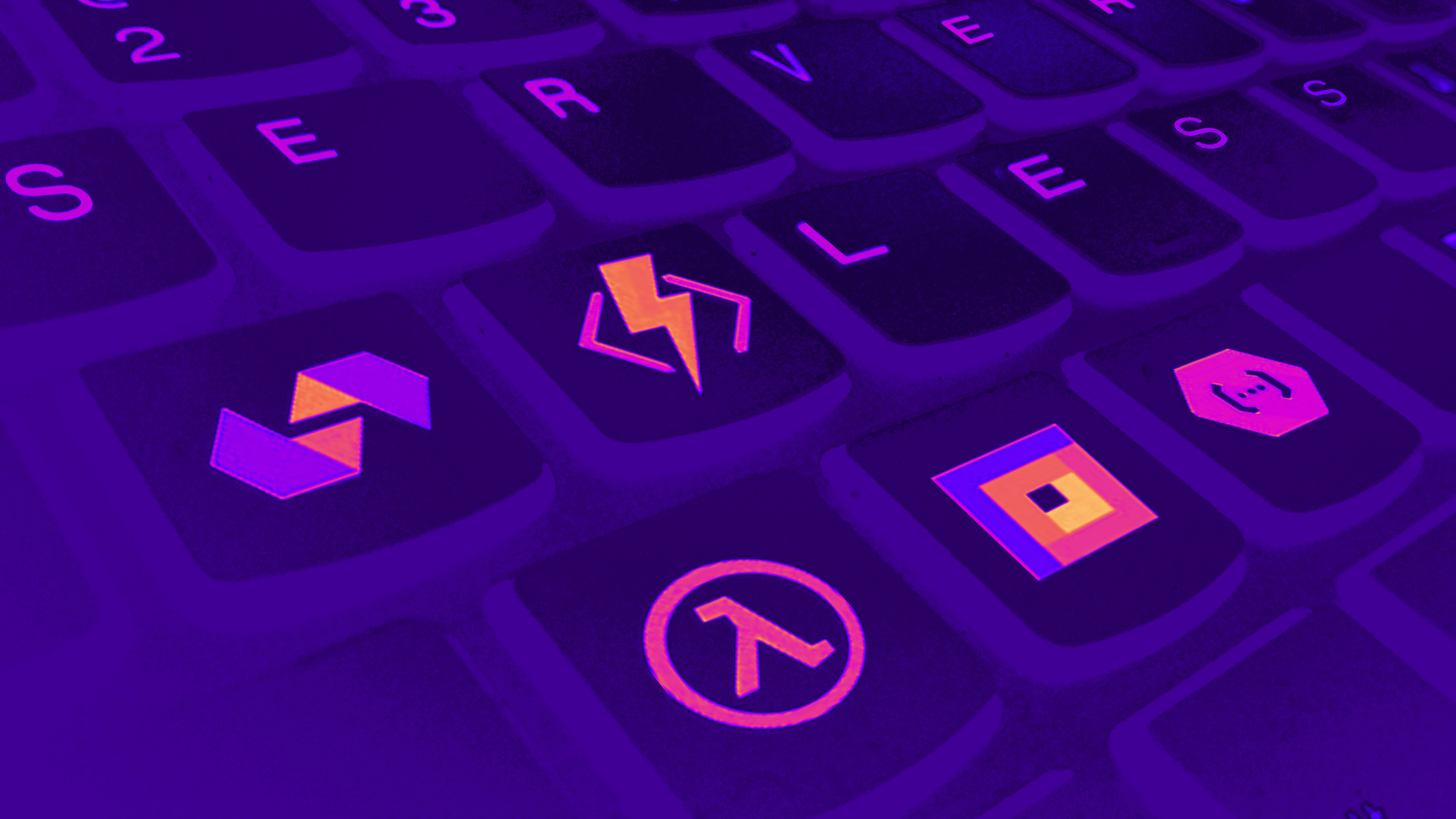
In today's fast-paced technological landscape, businesses are constantly seeking ways to improve the efficiency and scalability of their applications. Microservices architecture has emerged as a popular approach, breaking down monolithic applications into smaller, manageable services.
Alongside this, serverless computing has gained traction for its ability to run functions in the cloud without the need to manage infrastructure.
Integrating serverless functions with existing microservices can provide a powerful combination of scalability, cost-effectiveness, and rapid deployment. This article explores how to achieve this integration effectively.
Understanding Serverless Functions
Serverless functions, often referred to as Functions-as-a-Service (FaaS), are small pieces of code that execute in response to events and automatically scale with demand. AWS Lambda, Azure Functions, and Google Cloud Functions are popular examples of serverless platforms. These functions are stateless, ephemeral, and managed by cloud providers, allowing developers to focus solely on writing code.
Benefits of Integrating Serverless Functions with Microservices
- Scalability: Serverless functions automatically scale with demand, ensuring that your microservices can handle varying workloads without manual intervention.
- Cost Efficiency: With a pay-as-you-go pricing model, serverless functions can reduce costs by only charging for the actual execution time and resources used.
- Rapid Development: Serverless functions enable faster development cycles by abstracting infrastructure management, allowing teams to deploy new features quickly.
- Event-Driven Architecture: Serverless functions excel in event-driven scenarios, complementing microservices that often rely on events for inter-service communication.
Integration Strategies
1. Event-Driven Integration
In an event-driven architecture, services communicate through events. Serverless functions can be seamlessly integrated into this architecture by acting as event processors.
Steps to Implement:
- Identify Events: Determine the events that your microservices produce and consume.
- Set Up Event Sources: Configure event sources such as AWS SNS, Azure Event Grid, or Google Pub/Sub.
- Create Serverless Functions: Write serverless functions to handle specific events. For example, an AWS Lambda function can be triggered by an SNS topic.
- Connect Microservices: Modify your microservices to publish events to the chosen event source, triggering the serverless functions.
Example:
import boto3
sns = boto3.client('sns')
def publish_event(message):
sns.publish(
TopicArn='arn:aws:sns:us-east-1:123456789012:MyTopic',
Message=message
)
2. API Gateway Integration
Serverless functions can extend the capabilities of your microservices by acting as API endpoints. This is particularly useful for adding new features without modifying the existing microservice codebase.
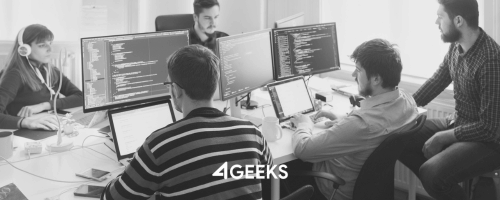
Custom Software Engineering Services
Work with our in-house Project Managers, Software Engineers and QA Testers to build your new custom software product or to support your current workflow, following Agile, DevOps and Lean methodologies.
Steps to Implement:
- Create an API Gateway: Use services like AWS API Gateway or Azure API Management to create and manage APIs.
- Define Routes: Set up routes in the API gateway that map to serverless functions.
- Write Serverless Functions: Implement the serverless functions that will handle requests to the new API endpoints.
- Integrate with Microservices: Ensure the serverless functions can communicate with your microservices, either directly via HTTP calls or through a message broker.
Example:
exports.handler = async (event) => {
const response = await fetch('https://api.microservice.com/data', {
method: 'GET'
});
const data = await response.json();
return {
statusCode: 200,
body: JSON.stringify(data)
};
};
3. Middleware and Shared Logic
Serverless functions can also serve as middleware to offload common tasks such as authentication, logging, and data transformation.
Steps to Implement:
- Identify Common Logic: Determine tasks that can be centralized into serverless functions.
- Write Serverless Functions: Develop the functions to handle these tasks.
- Modify Microservices: Update your microservices to call these serverless functions when performing the identified tasks.
Example:
def lambda_handler(event, context):
auth_token = event['headers'].get('Authorization')
if not validate_token(auth_token):
return {
'statusCode': 403,
'body': 'Forbidden'
}
# Proceed with the main logic
...
4. Data Processing
Serverless functions are ideal for data processing tasks, such as ETL (Extract, Transform, Load) operations. Integrate them to handle data processing workloads that complement your microservices.
Steps to Implement:
- Identify Data Processing Needs: Determine which data processing tasks can be offloaded to serverless functions.
- Create Data Processing Functions: Develop serverless functions for tasks such as data transformation or aggregation.
- Integrate Data Pipelines: Ensure data flows seamlessly between your microservices and the serverless functions, using services like AWS S3, Azure Blob Storage, or Google Cloud Storage.
Example:
def lambda_handler(event, context):
for record in event['Records']:
data = transform_data(record['body'])
store_data(data)
def transform_data(data):
# Transform the data as needed
return transformed_data
def store_data(data):
# Store the data in a database or another storage service
...
Best Practices
- Modular Design: Keep serverless functions focused on single tasks to ensure modularity and ease of maintenance.
- Security: Implement robust security practices, such as least privilege access, encryption, and API authentication.
- Monitoring and Logging: Use cloud provider tools like AWS CloudWatch, Azure Monitor, or Google Cloud Logging to monitor and log serverless functions.
- Testing: Thoroughly test serverless functions in isolation and as part of the integrated system to ensure reliability.
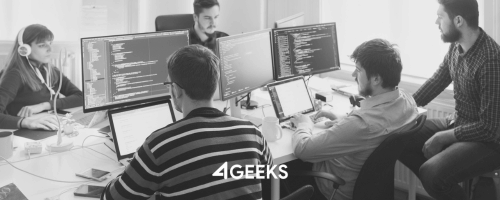
Custom Software Engineering Services
Work with our in-house Project Managers, Software Engineers and QA Testers to build your new custom software product or to support your current workflow, following Agile, DevOps and Lean methodologies.
Conclusion
Integrating serverless functions with existing microservices can significantly enhance the scalability, cost-efficiency, and agility of your applications. By adopting strategies such as event-driven integration, API gateway integration, middleware, and data processing, you can leverage the strengths of both architectures.
Embrace best practices to ensure a secure, maintainable, and performant system. As cloud technologies continue to evolve, this hybrid approach will position your applications for future success.
FAQs
What are some potential challenges when integrating serverless functions with microservices?
Potential challenges include managing the increased complexity of having more distributed components, ensuring consistent security practices across microservices and serverless functions, handling state management in a stateless environment, and dealing with the latency introduced by network calls between services.
How can serverless functions interact with stateful microservices that require maintaining state?
Serverless functions can interact with stateful microservices by using external state management solutions such as databases, caching systems (e.g., Redis), or stateful services provided by the cloud provider. For instance, AWS Step Functions can orchestrate serverless workflows while maintaining state.
What are some common use cases where integrating serverless functions with microservices is particularly beneficial?
Common use cases include real-time data processing (e.g., IoT data ingestion), event-driven applications (e.g., user notifications), extending microservices with new features without changing the existing codebase (e.g., adding new API endpoints), and offloading background tasks (e.g., image processing or batch data transformation).